WordPress has always been a go-to platform for anyone looking to build a website with minimal fuss, but what about the bits under the hood that drive real-time interactions?
For years, adding interactivity meant relying on external scripts or plugins. With the Interactivity API, WordPress has a native solution that simplifies the process, allowing developers to focus on creating smooth user experiences.
It’s not a huge shift, but it’s the kind of under-the-surface update that addresses a real need – letting websites feel more alive, without the overhead of extra tools.
If you’re building in WordPress, this is something worth paying attention to – let’s get you started.
What is the WordPress Interactivity API, and why do developers need it?
The WordPress Interactivity API is a built-in tool that lets you add real-time, dynamic features to your website. With it, you can update parts of a page, like notifications or content changes, instantly without needing to refresh the whole page. This makes your site feel more responsive and interactive for users.
Before this API, adding these kinds of interactive features often required using third-party tools or custom JavaScript, which could slow down your site or cause compatibility issues.
For example, you might have used something like React to manage real-time content. While powerful, React and similar tools can be complex and add extra load to your site. The Interactivity API solves this by providing a simpler, faster way to handle updates directly within WordPress, without needing extra libraries or plugins.
If you’re a WordPress developer, you’ll be glad to know that the API:
- Improves performance by allowing developers to update only the necessary parts of a page. This eliminates full page reloads and minimizes the need for heavy external scripts, making sites faster and more responsive.
- Simplifies development by offering a native, standardized solution. You can avoid custom JavaScript and third-party libraries, cutting down on complexity and minimizing compatibility issues.
- Increases scalability by providing reusable functions for dynamic content. You can easily add interactive features across multiple sites without creating custom solutions each time.
- Enhances compatibility by being built directly into WordPress. The API integrates seamlessly with the platform, reducing conflicts with updates or other plugins.
- Promotes better site maintenance by reducing dependency on external tools. Since it’s native to WordPress, developers can rely on a stable, long-term solution with fewer troubleshooting needs.
Prerequisites for getting started with the WordPress Interactivity API
To get started with the Interactivity API, you’ll need:
- Basic WordPress development knowledge for working with themes, plugins, and WordPress hooks. Without it, you may face compatibility issues and find it difficult to make the features work properly.
- WordPress Version 6.5 or later, as that’s the version that introduced the API. If you’re on an earlier version, you’ll need to rely on alternative methods for dynamic content.
- JavaScript and AJAX knowledge for handling dynamic content updates and real-time interactions. Without this, implementing interactive features can become difficult, and troubleshooting may be time-consuming.
- Understanding how to enqueue scripts for managing JavaScript properly in WordPress. Otherwise, your scripts may fail to load correctly, causing performance issues or broken functionality.
- Familiarity with WordPress’s REST API for managing real-time data updates. Without this, fetching and updating dynamic data can become challenging, leading to incomplete or broken interactive features.
- A development environment for testing changes before applying them to your live site. If you don’t use one, you risk causing errors or downtime on your live site.
Comparing approaches: Why the Interactivity API can replace complex JavaScript frameworks
The WordPress Interactivity API provides a simpler and more integrated way to add dynamic content, which can often replace the need for complex JavaScript frameworks like React or Vue.
For example, if you want to display live comments or real-time notifications, you can use the Interactivity API to update those elements dynamically without reloading the entire page.
With React or Vue, these features would typically require setting up a full JavaScript-based frontend with complex state management and integration with WordPress via AJAX or a custom REST API endpoint. This often results in increased load times and more complex code.
Unlike external APIs, the Interactivity API simplifies this process by offering built-in functions to update specific parts of the page, such as a live feed or form submission, without needing to load large JavaScript libraries or manage state on the frontend.
For instance, a feature like live form validation – updating the form as the user types – can be implemented directly with the API, avoiding the need for an external framework.
In contrast, React might be more suitable for a single-page application or complex UIs where the state needs to be shared across multiple components.
However, for most WordPress sites, especially those with simpler dynamic features like real-time post updates or instant content changes, the Interactivity API provides a more efficient and streamlined approach that’s fully integrated with the WordPress ecosystem.
Understanding Directives and Store
In the context of the WordPress Interactivity API, directives are instructions that tell the system how to update specific parts of a page in response to user interactions.
They define which elements of the page should be modified when certain actions occur, like a form submission or a content update. Directives are written in JavaScript and are linked to specific parts of the page using identifiers, allowing for dynamic updates without reloading the whole page.
The store in the Interactivity API manages the state of the page. It stores data such as form inputs, user selections, or any other dynamic content that needs to persist while interacting with the page.
The store ensures that changes to the content are reflected across the page, making it easier to maintain state consistency as users interact with the site.
Practical implementation: Building real-world interactive components
Ready to dip your toes in? We’re going to walk you through a simple but realistic use case for the Interactivity API: a button that increments by one on click and outputs the number of clicks.
You can add this functionality as part of a custom plugin:
- In wp-content/plugins create a folder called simple-click-counter.
- In this folder, add a file called simple_click_counter.php that registers a custom counter block and also makes it accessible via a shortcode for more flexibility.
- Paste the following code into this file:
<?php
/**
* Plugin Name: Simple Click Counter
* Description: A minimal click counter using WordPress Interactivity API
* Version: 1.0.0
*/
// Register the block
function register_click_counter_block() {
// Register script
wp_register_script(
'click-counter-script',
plugins_url('counter.js', __FILE__),
array('wp-interactivity'),
'1.0.0',
true
);
// Register block
register_block_type('click-counter/block', array(
'render_callback' => 'render_click_counter_block',
'view_script_module' => 'click-counter-script',
'supports' => array(
'interactivity' => true,
),
));
}
add_action('init', 'register_click_counter_block');
// Render the block
function render_click_counter_block() {
ob_start();
?>
<div <?php echo get_block_wrapper_attributes(array(
'data-wp-interactive' => 'click-counter',
'data-wp-context' => wp_json_encode(array('count' => 0)),
)); ?>>
<button data-wp-on--click="actions.increment">Click here</button>
<div>
Clicks: <span data-wp-text="context.count">0</span>
</div>
</div>
<?php
return ob_get_clean();
}
// Register shortcode for non-block editor usage
function click_counter_shortcode() {
return render_click_counter_block();
}
add_shortcode('click_counter', 'click_counter_shortcode');
- Still in the same folder, create a file called counter.js, which is referenced in the code above and contains the actual increment functionality. Add the following code to it:
import { store, getContext } from '@wordpress/interactivity';
store('click-counter', {
actions: {
increment: () => {
const context = getContext();
context.count += 1;
},
},
});
- From your admin dashboard, go to Plugins > Installed Plugins and activate the custom plugin.

- Open your editor, e.g., by creating a new post or page, and add the block using the shortcode [click_counter].
- Publish the content and load it up on the frontend, where you can test its functionality.
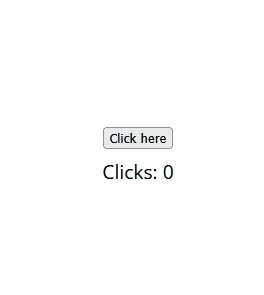
It’s simple, sure, but think of what you could do by building on the concept, from displaying cart items to social interactions like likes and shares in real time!
Need help implementing the Interactivity API? Hire Codeable’s WordPress experts
The WordPress Interactivity API does a lot to bring the platform into the future, but it’s still out of reach for any non-advanced users.
If you recognize its potential but can’t or don’t want to implement it yourself, consider hiring a WordPress developer through Codeable.
Our vetted experts have years of real-world experience that you can bring on board for your project without the hassle of sorting through endless applications and proposals. They’ve also specialized in WordPress development, meaning you skip the trial-and-error phase of working with someone who isn’t.
When you’re ready to take your site’s user experience to the next level with the WordPress interactivity API, submit your first project to Codeable!